What are Functions in Python? A Comprehensive Guide
Functions are one of the most important concepts in Python and programming in general. They allow you to encapsulate a block of code that performs a specific task, making your programs more modular, reusable, and easier to debug. In this blog, we’ll explore what functions are, how to define and use them, and some advanced concepts like arguments, return values, and lambda functions.
1. What is a Function?
A function is a block of organized, reusable code that performs a single, related action. Functions provide better modularity for your application and a high degree of code reusability. In Python, you can define a function using the def
keyword.
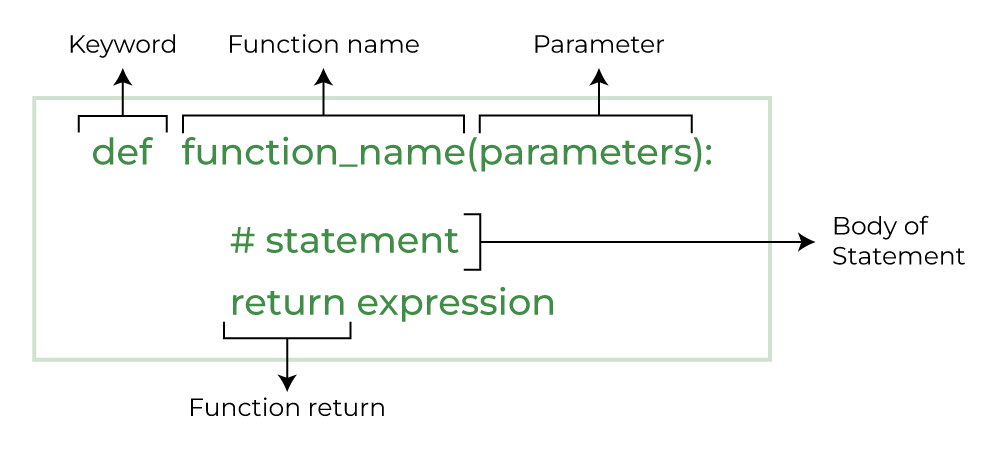
# Basic function example
def greet():
print("Hello, World!")
Flow Explanation: The function greet()
is defined to print "Hello, World!". To execute the function, you need to call it by writing greet()
.
2. Function Arguments
Functions can accept inputs, called arguments, which allow you to pass data into the function. This makes functions more flexible and reusable.
# Function with arguments
def greet(name):
print(f"Hello, {name}!")
Flow Explanation: The function greet()
now takes an argument name
. When you call greet("Alice")
, it prints "Hello, Alice!".
3. Return Values
Functions can also return values using the return
statement. This allows you to use the result of a function in other parts of your program.
# Function with return value
def add(a, b):
return a + b
Flow Explanation: The function add()
takes two arguments, adds them, and returns the result. You can store the result in a variable or use it directly.
4. Default Arguments
Python allows you to define default values for arguments. If the caller doesn’t provide a value for that argument, the default value is used.
# Function with default argument
def greet(name="Guest"):
print(f"Hello, {name}!")
Flow Explanation: If you call greet()
without an argument, it uses the default value "Guest"
and prints "Hello, Guest!".
5. Lambda Functions
Lambda functions are small, anonymous functions defined using the lambda
keyword. They are useful for short, throwaway functions.
# Lambda function example
square = lambda x: x ** 2
print(square(5)) # Output: 25
Flow Explanation: The lambda function square
takes one argument x
and returns its square. It’s a concise way to define simple functions.
6. Fun Example: Temperature Converter
Let’s create a function to convert Celsius to Fahrenheit.
# Temperature converter function
def celsius_to_fahrenheit(celsius):
return (celsius * 9/5) + 32
print(celsius_to_fahrenheit(25)) # Output: 77.0
Flow Explanation: The function celsius_to_fahrenheit()
takes a temperature in Celsius and returns the equivalent temperature in Fahrenheit.
Complete Example: Calculate Area of a Rectangle
Let’s create a function to calculate the area of a rectangle. This example demonstrates how to define a function, pass arguments, and return a value.
# Function to calculate the area of a rectangle
def calculate_area(length, width):
"""
This function calculates the area of a rectangle.
Parameters:
length (float): The length of the rectangle.
width (float): The width of the rectangle.
Returns:
float: The area of the rectangle.
"""
area = length * width
return area
# Example usage
length = 5.0
width = 3.0
result = calculate_area(length, width)
print(f"The area of the rectangle is: {result}")
Flow Explanation:
- The function
calculate_area()
takes two arguments:length
andwidth
. - It calculates the area by multiplying the length and width.
- The result is returned and stored in the variable
result
. - Finally, the area is printed using an f-string.
Conclusion
Functions are a cornerstone of Python programming. They help you write clean, reusable, and modular code. By mastering functions, arguments, return values, and lambda functions, you can significantly improve your coding efficiency. Start experimenting with functions in your projects and see how they can simplify your code!
0 Comments